I had a scenario in electronic report where I wanted to reuse existing X++ code that generates some temporary table records for reporting purpose. Therefore I wanted electronic reporting to call my X++ method (for a particular context, an invoice in this case) and use the list of records returned by this method.
Electronic reporting supports method calls, but all the information I found on internet was about methods returning a single primitive value or a single record.
But it turned out it’s supported and actually quite easy to use.
The key is that the method must return on of the supported data types and it must be decorated with ERTableNameAttribute. Like this:
[ERTableName(tableStr(MyTable))] public static RecordLinkList getData() { ... }
The supported data types are:
- Query
- RecordLinkList
- RecordSortedList
- any class implementing System.Collections.IEnumerable interface (.NET arrays, lists etc.)
If you wonder how I know it, I found the definition in ERDataContainerDescriptorBase.isRecordCollection().
One way of using such a method is defining a static method in class and using it the Class data source in ER.
Let me also extend the example with a parameter, to give the method some instructions about what it should generate:
public class ERTableDemo { [ERTableName(tableStr(MyTable))] public static RecordLinkList getData(str _param) { ... } }
In a model mapping, add a new Class data source and add your class. You’ll see a list of methods and if you expand the method, you’ll see the table fields available for binding.
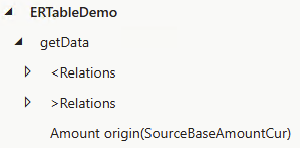
When binding the method to a record list, we can provide a value for the parameter. We also can bind field values as usual:

But I would rather use an instance method on a table, which would produce data related to the given record (such as an invoice).
I saw instance methods with ERTableNameAttribute in standard code, therefore I knew it can be done on tables, but I wasn’t sure that ER takes table extensions into account.
I tried an extension like this:
[ExtensionOf(tableStr(CustInvoiceJour))] public final class CustInvoiceJourMy_Extension { [ERTableName(tableStr(MyTable))] public RecordLinkList getMyTableRecords() { ... } }
and I am able to use it in ER model mapping:

This is ideal.